Enhance your Python skills using Python coding exercises and provide hands-on experience with clear, concise code snippets. Whether you’re a beginner or looking to refine your coding expertise, these exercises are crafted to empower you with practical knowledge.
You can run the code below using the online Integrated Development environment- Replit or by downloading one on your system- Python download
Python coding Exercises: Integer to Character
Unlock the secrets of converting ASCII values to characters in Python effortlessly. Explore the simple yet powerful code snippets.
num = int(input("Enter an ASCII value: "))
print("%c" % num)
Output:
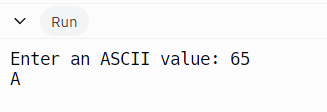
Python Coding Exercises: Decimal Places
Master the art of handling decimal places in Python. Get hands-on experience with code that lets you control precision.
num = float(input("Enter a Number: "))
print("{:.2f}".format(num))
Output
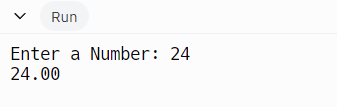
Python Tuple Assignment
Efficiently assign multiple variables in a single statement using Python’s tuple assignment. Enhance your coding skills with concise and powerful techniques.
num1, num2, name = 101, 102, 'John'
print("{} {} {}".format(num1, num2, name))
Output

Python Decimal to Hexadecimal
Delve into the hexadecimal world with Python. Learn to convert decimal numbers to their hexadecimal counterparts effortlessly.
num = int(input("Enter a Number: "))
print("{:x}".format(num))
Output

Python Hexadecimal to Decimal
Uncover the mysteries of hexadecimal literals in Python and effortlessly convert them to decimal representation.
num = 0xb
print(num)
Output
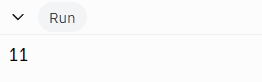
Python Exponent Representation
Experience the power of exponent representation in Python. Learn to display numbers in a concise and informative format.
price = float(input("Enter the cost: "))
print("%E" % price)
Output
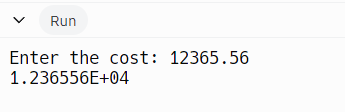
>>Webinar